SOLID PRINCIPLES IN JAVA
SOLID principles are the foundation of object-oriented programming. Explore how these principles apply in Java to create robust and flexible software.
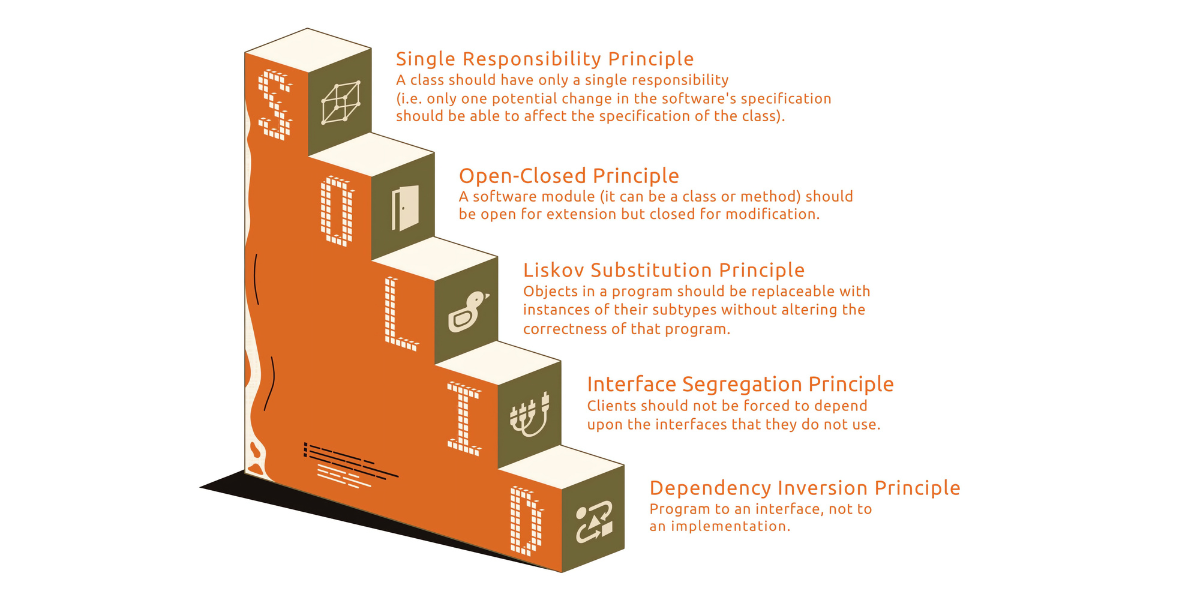
SOLID principles are a set of design guidelines that help developers create software that is easy to understand, extend, and maintain. By adhering to these principles, Java developers can avoid code smells and create robust applications.
1. Single Responsibility Principle (SRP)
The Single Responsibility Principle states that a class should have only one reason to change. This means each class should only perform one specific task.
Implementation in Java:
- Break down large classes into smaller, focused classes.
- Ensure each class is responsible for a single functionality.
Example: A class handling user authentication should not also manage logging or database connections.
2. Open/Closed Principle (OCP)
The Open/Closed Principle states that software entities should be open for extension but closed for modification. In Java, this is often achieved using interfaces and abstract classes.
Implementation in Java:
- Use inheritance and polymorphism to extend functionality.
- Avoid changing existing code when adding new features.
Example: Adding new types of payment methods without modifying the existing payment processing code.
3. Liskov Substitution Principle (LSP)
The Liskov Substitution Principle states that objects of a superclass should be replaceable with objects of a subclass without affecting the functionality of the program.
Implementation in Java:
- Ensure subclasses do not violate the behavior of the parent class.
- Test polymorphic behavior thoroughly.
Example: A method expecting a base class type should work seamlessly when passed a derived class instance.
4. Interface Segregation Principle (ISP)
The Interface Segregation Principle states that a class should not be forced to implement interfaces it does not use. Instead, create smaller, more specific interfaces.
Implementation in Java:
- Split large interfaces into smaller ones.
- Use multiple specific interfaces for different functionalities.
Example: A "Printer" interface should not force a class to implement both print and scan functionalities if it only prints.
5. Dependency Inversion Principle (DIP)
The Dependency Inversion Principle states that high-level modules should not depend on low-level modules; both should depend on abstractions. This promotes loose coupling.
Implementation in Java:
- Depend on interfaces or abstract classes, not concrete implementations.
- Use dependency injection frameworks like Spring to manage dependencies.
Example: A service class depends on an abstraction of a repository interface rather than a specific database implementation.
Technology Insights
Java's robust features, such as interfaces, abstract classes, and dependency injection frameworks like Spring, make it an ideal language to implement SOLID principles effectively, ensuring clean and maintainable code.